Post Stastics
- This post has 1160 words.
- Estimated read time is 5.52 minute(s).
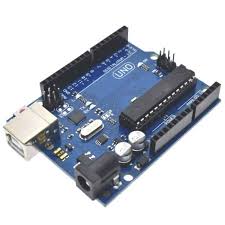
Introduction
The Arduino platform has revolutionized the way hobbyists, engineers, and students engage with electronics and embedded systems. Its simplicity and user-friendly environment make it an excellent entry point for learning the C programming language. While the Arduino IDE abstracts much of the complexity away, understanding the underlying C language provides you with more control and flexibility, enabling you to write more efficient and complex programs.
In this article, we’ll dive into the C language, specifically focusing on how it’s used in the Arduino environment. We’ll explore key concepts such as variables, data types, control structures, functions, and pointers. Each section includes practical examples that you can try on your Arduino board.
Getting Started: Understanding the Basics
Before we start coding, it’s important to understand that C is a procedural programming language. This means that C programs are a sequence of instructions that the processor executes. The structure of a typical C program consists of:
- Preprocessor Directives: These are commands that are processed before the actual compilation of code begins.
- Functions: Blocks of code that perform specific tasks.
- Variables: Storage locations for data.
- Statements and Expressions: The instructions that perform operations.
Example: Basic Structure of a C Program
#include <Arduino.h> void setup() { // Initialize serial communication at 9600 bps Serial.begin(9600); } void loop() { // Print a message to the serial monitor Serial.println("Hello, World!"); delay(1000); // Wait for 1 second }
In this example, setup()
and loop()
are functions that structure your Arduino program. The setup()
function runs once when you power up or reset your Arduino board, and the loop()
function runs continuously.
Variables and Data Types
In C, a variable is a named location in memory that stores a value. Each variable has a specific data type, which determines the size and layout of the variable’s memory. The most commonly used data types in Arduino programming are:
- int: Used to store integers.
- float: Used to store decimal numbers.
- char: Used to store characters.
- boolean: Used to store
true
orfalse
.
Example: Using Variables
int ledPin = 13; // Pin 13 has an LED connected on most Arduino boards int delayTime = 500; // Time delay in milliseconds void setup() { pinMode(ledPin, OUTPUT); // Set the LED pin as an output } void loop() { digitalWrite(ledPin, HIGH); // Turn the LED on delay(delayTime); // Wait for delayTime milliseconds digitalWrite(ledPin, LOW); // Turn the LED off delay(delayTime); // Wait for delayTime milliseconds }
In this example, ledPin
and delayTime
are variables that store the pin number and delay time, respectively. The int
keyword indicates that these variables hold integer values.
Operators
Operators in C allow you to perform operations on variables and values. There are several types of operators:
- Arithmetic Operators:
+
,-
,*
,/
,%
- Comparison Operators:
==
,!=
,<
,>
,<=
,>=
- Logical Operators:
&&
,||
,!
- Assignment Operators:
=
,+=
,-=
,*=
,/=
,%=
Example: Using Operators
int x = 10; int y = 5; int z; void setup() { Serial.begin(9600); z = x + y; // Addition Serial.println(z); // Prints 15 z = x - y; // Subtraction Serial.println(z); // Prints 5 z = x * y; // Multiplication Serial.println(z); // Prints 50 z = x / y; // Division Serial.println(z); // Prints 2 z = x % y; // Modulus Serial.println(z); // Prints 0 } void loop() { // Empty loop }
In this code, we perform basic arithmetic operations using variables x
and y
, and store the results in variable z
.
Control Structures
Control structures allow you to control the flow of your program. The most common control structures are:
- if-else: Conditional statements that execute code based on a condition.
- for: Loops that execute a block of code a specific number of times.
- while: Loops that execute a block of code as long as a condition is true.
- switch-case: A multi-way branch statement that executes one block of code among many.
Example: Using Control Structures
int sensorValue; int threshold = 500; void setup() { Serial.begin(9600); pinMode(13, OUTPUT); } void loop() { sensorValue = analogRead(A0); // Read the sensor value from analog pin A0 if (sensorValue > threshold) { digitalWrite(13, HIGH); // Turn on the LED if sensor value exceeds threshold } else { digitalWrite(13, LOW); // Turn off the LED otherwise } delay(100); }
Here, we use an if-else
statement to turn an LED on or off based on the value read from an analog sensor.
Functions
Functions in C are reusable blocks of code that perform a specific task. They help in organizing code and reducing redundancy.
Example: Creating and Using Functions
int ledPin = 13; void setup() { pinMode(ledPin, OUTPUT); } void loop() { blinkLED(ledPin, 3); // Blink the LED 3 times delay(2000); // Wait for 2 seconds before repeating } void blinkLED(int pin, int times) { for (int i = 0; i < times; i++) { digitalWrite(pin, HIGH); delay(500); digitalWrite(pin, LOW); delay(500); } }
In this example, the blinkLED()
function blinks an LED a specified number of times. By defining this behavior as a function, we can easily reuse it throughout the program.
Arrays
An array in C is a collection of variables of the same type. Arrays are useful for storing lists of data.
Example: Working with Arrays
int ledPins[] = {2, 3, 4, 5}; // Array of LED pins void setup() { for (int i = 0; i < 4; i++) { pinMode(ledPins[i], OUTPUT); // Set each pin in the array as an output } } void loop() { for (int i = 0; i < 4; i++) { digitalWrite(ledPins[i], HIGH); // Turn on each LED delay(500); digitalWrite(ledPins[i], LOW); // Turn off each LED delay(500); } }
In this code, ledPins
is an array that holds the pin numbers of four LEDs. The for
loop iterates through the array to turn each LED on and off.
Pointers
Pointers are variables that store the memory address of another variable. They are a powerful feature of C, allowing for dynamic memory allocation, efficient array handling, and more.
Example: Introduction to Pointers
int value = 10; int *ptr; void setup() { Serial.begin(9600); ptr = &value; // Assign the address of value to ptr Serial.println(*ptr); // Dereference ptr to get the value at the address } void loop() { // Empty loop }
In this example, ptr
is a pointer that holds the address of the variable value
. The expression *ptr
dereferences the pointer, giving us the value stored at that address.
Advanced Topics: Structs and Unions
As you become more comfortable with C, you’ll encounter more advanced features like structs and unions, which allow you to create complex data structures.
- Structs: Used to group variables of different types together.
- Unions: Similar to structs, but members share the same memory location.
Example: Using Structs
struct SensorData { int sensorValue; float voltage; }; void setup() { Serial.begin(9600); SensorData data; data.sensorValue = analogRead(A0); data.voltage = (data.sensorValue / 1023.0) * 5.0; Serial.println(data.voltage); } void loop() { // Empty loop }
In this code, SensorData
is a struct that groups a sensor value and its corresponding voltage.
Conclusion
C is a powerful language that forms the backbone of Arduino programming. While the Arduino IDE simplifies many aspects, understanding C allows you to harness the full potential of the platform. This introduction has covered the essentials, but there’s much more to explore, including libraries, memory management, and optimization techniques.
Experiment with the examples provided, modify them, and see how small changes affect the behavior of your Arduino. The best way to learn is by doing, so keep coding and tinkering with your Arduino projects.