Post Stastics
- This post has 1653 words.
- Estimated read time is 7.87 minute(s).
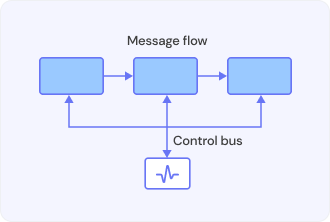
A Beginners Guide
Introduction
Communication protocols are vital for enabling embedded devices to interact effectively. Three prominent protocols, Modbus, Standard Commands for Programmable Instruments (SCPI), and General Purpose Interface Bus (GPIB), stand out due to their widespread adoption in various industrial and testing environments. Recently, I had the opportunity to work on an embedded project developing a piece of environmental test equipment for electronics testing, where Modbus, SCPI, and GPIB protocols played crucial roles.
This article delves into the specifics of these protocols, explaining their functionalities and detailing how to implement them on microcontrollers using Arduino and MicroPython. By the end, you should have a comprehensive understanding of these protocols and practical knowledge to deploy them in your projects.
Why Modbus, SCPI, and GPIB Protocols Exist
The Need for Communication Protocols
Communication protocols are essential for establishing standardized methods of data exchange between devices. They ensure interoperability, reliability, and efficiency in transmitting information, which is crucial for automation, control, and testing applications. Without standardized protocols, devices from different manufacturers would struggle to communicate effectively, leading to inefficiencies and increased complexity in system design.
Where Modbus, SCPI, and GPIB Fit In
- Modbus: Primarily used in industrial automation and control systems. It facilitates communication between various types of equipment, such as sensors, actuators, and controllers, over a common network.
- SCPI: Focused on controlling and communicating with test and measurement instruments. It standardizes the commands for instrument configuration, measurement, and data acquisition.
- GPIB: An older standard, primarily used for connecting and controlling multiple instruments and computers. GPIB provides a robust and reliable interface for complex setups in laboratories and industrial environments.
Histories
Modbus
- Developed By: Modicon (now Schneider Electric) in 1979.
- Purpose: Designed to facilitate communication between programmable logic controllers (PLCs) and other industrial devices.
- Evolution: Initially implemented over serial communication (RS-232 and RS-485) and later extended to Ethernet (Modbus TCP), and has been used over USB.
SCPI
- Developed By: Introduced by the SCPI Consortium in 1990.
- Purpose: Created to standardize the control of test and measurement instruments, ensuring consistency across different manufacturers.
- Evolution: Built upon the IEEE 488.2 standard, SCPI provided a common set of commands for a wide range of instruments.
GPIB
- Developed By: Originally developed by Hewlett-Packard (HP) in the 1960s as the Hewlett-Packard Interface Bus (HP-IB).
- Purpose: Designed to connect and control multiple instruments and computers in laboratory and industrial environments.
- Evolution: Standardized as IEEE 488 in 1975, GPIB became a widely adopted interface for instrument control before being largely superseded by more modern interfaces like USB and Ethernet.
Key Differences and Use Cases
Modbus
- Architecture: Uses a master-slave architecture where one master device controls multiple slave devices.
- Data Transmission: Supports serial (Modbus RTU and ASCII) and network-based (Modbus TCP) communication.
- Use Cases: Ideal for industrial environments where reliable, real-time communication between various control and monitoring devices is essential.
SCPI
- Command Structure: SCPI commands are text-based and follow a hierarchical structure, making them human-readable and easy to understand.
- Focus: Designed specifically for controlling programmable instruments and obtaining measurement data.
- Use Cases: Perfect for laboratory and testing environments where precise control and standardized communication with measurement instruments are required.
GPIB
- Connectivity: Provides a robust, parallel communication interface capable of connecting multiple devices in a daisy-chain configuration.
- Standardization: Widely adopted as IEEE 488, ensuring compatibility across various instruments and manufacturers.
- Use Cases: Suitable for complex laboratory setups and industrial environments where multiple instruments need to be controlled and monitored simultaneously.
Implementing Modbus on Microcontrollers
Arduino Example
To implement Modbus on an Arduino, we can use the “SimpleModbus” library. Below is a basic example of setting up an Arduino as a Modbus slave.
#include <SimpleModbusSlave.h> #define LED_PIN 13 // Modbus Registers Offsets (0-9999) const int regLedState = 0; // Modbus Registers Array unsigned int holdingRegs[1]; void setup() { // Pin initialization pinMode(LED_PIN, OUTPUT); // Modbus setup modbus_configure(&Serial, 9600, SERIAL_8N1, 1, 2, holdingRegs, sizeof(holdingRegs)/sizeof(holdingRegs[0])); // Set initial LED state digitalWrite(LED_PIN, holdingRegs[regLedState]); } void loop() { // Modbus loop modbus_update(); // Update LED state based on Modbus register digitalWrite(LED_PIN, holdingRegs[regLedState]); }
In this example, the Arduino listens for Modbus commands on the Serial port and controls an LED based on the value of a holding register.
MicroPython Example
For MicroPython, we use the “umodbus” library to implement Modbus functionality on a microcontroller such as the ESP8266 or ESP32.
from machine import Pin, UART import uModBus.serial as modbus # Initialize UART and Modbus RTU uart = UART(1, baudrate=9600, tx=17, rx=16) mb = modbus.ModbusRTU(uart, 1) # LED Pin led = Pin(2, Pin.OUT) # Holding Register holding_registers = [0] # Function to handle Modbus requests def handle_modbus(): global holding_registers request = uart.read() if request: response = mb.handle(request, holding_registers=holding_registers) uart.write(response) led.value(holding_registers[0]) while True: handle_modbus()
This MicroPython example sets up a Modbus RTU slave that controls an LED based on the value received in the holding register.
Understanding SCPI Protocol
Overview of SCPI
SCPI (Standard Commands for Programmable Instruments) is a standard command set for controlling programmable instruments. Developed in the late 1980s, SCPI commands are textual and often used over interfaces like GPIB, USB, and Ethernet to enable communication with test and measurement devices.
Key Features of SCPI
- Standardization: Provides a common language for instrument control.
- Hierarchical Structure: Commands are organized in a tree structure for easy navigation.
- Flexibility: Supports a wide range of instruments and functionalities.
- Extensibility: Allows manufacturers to add custom commands while maintaining compatibility.
SCPI Command Structure
SCPI commands are human-readable text strings, organized into a hierarchical structure. They typically follow the format:
[<Subsystem>:]<Command>[<Space><Parameters>]
For example, the command MEAS:VOLT:DC?
queries the DC voltage measurement.
Implementing SCPI on Microcontrollers
Arduino Example
To implement SCPI on an Arduino, we can use a simple serial communication approach. Below is an example of an Arduino SCPI implementation that responds to basic commands.
#include <Arduino.h> #define LED_PIN 13 void setup() { Serial.begin(9600); pinMode(LED_PIN, OUTPUT); Serial.println("SCPI Device Ready"); } void loop() { if (Serial.available()) { String command = Serial.readStringUntil('\n'); command.trim(); if (command == "*IDN?") { Serial.println("Arduino,SCPI Device,1.0"); } else if (command == "OUTP:STAT ON") { digitalWrite(LED_PIN, HIGH); Serial.println("LED ON"); } else if (command == "OUTP:STAT OFF") { digitalWrite(LED_PIN, LOW); Serial.println("LED OFF"); } else { Serial.println("Unknown Command"); } } }
In this example, the Arduino listens for SCPI commands on the Serial port and controls an LED accordingly.
MicroPython Example
For MicroPython, we use simple string parsing to implement SCPI functionality on a microcontroller such as the ESP8266 or ESP32.
from machine import Pin import sys # LED Pin led = Pin(2, Pin.OUT) def handle_scpi(command): command = command.strip() if command == "*IDN?": return "MicroPython,SCPI Device,1.0\n" elif command == "OUTP:STAT ON": led.value(1) return "LED ON\n" elif command == "OUTP:STAT OFF": led.value(0) return "LED OFF\n" else: return "Unknown Command\n" while True: if sys.stdin in select.select([sys.stdin], [], [], 0)[0]: command = sys.stdin.readline() response = handle_scpi(command) sys.stdout.write(response) sys.stdout.flush()
This MicroPython example reads SCPI commands from the standard input and controls an LED based on the received commands.
Understanding GPIB Protocol
Overview of GPIB
GPIB (General Purpose Interface Bus), also known as IEEE 488, is a standard interface for connecting and controlling multiple instruments and computers. It was developed by Hewlett-Packard (HP) in the 1960s and became a widely adopted standard for instrument control in laboratories and industrial environments.
Key Features of GPIB
- Robustness: Provides a reliable, parallel communication interface capable of connecting multiple devices.
- Standardization: Ensures compatibility across various instruments and manufacturers.
- Flexibility: Supports complex setups with multiple instruments controlled from a single interface.
GPIB Command Structure
GPIB commands are typically binary or ASCII-encoded strings sent over the bus to control instruments or retrieve data. The commands are similar to SCPI commands but are optimized for the GPIB interface.
Implementing GPIB on Microcontrollers
Implementing GPIB on microcontrollers can be more complex due to the hardware requirements for the GPIB interface. However, some specialized libraries and hardware modules are available to facilitate this implementation. Below is an example of how you might set up a basic GPIB interface using an Arduino with a GPIB shield.
Arduino Example
#include <Wire.h> #include <Arduino.h> #include <GPIB.h> void setup() { Serial.begin(9600); GPIB.begin(); Serial.println("GPIB Device Ready"); } void loop() { if (GPIB.available()) { String command = GPIB.readStringUntil('\n'); command.trim(); if (command == "*IDN?") { GPIB.println("Arduino,GPIB Device,1.0"); } else if (command == "OUTP:STAT ON") { digitalWrite(LED_PIN, HIGH); GPIB.println("LED ON"); } else if (command == "OUTP:STAT OFF") { digitalWrite(LED_PIN, LOW); GPIB.println("LED OFF"); } else { GPIB.println("Unknown Command"); } } }
This example sets up an Arduino to communicate over GPIB, responding to basic commands similar to the SCPI example.
Choosing Between Modbus, SCPI, and GPIB
When deciding which protocol to use, consider the following factors:
- Application Requirements: Modbus is ideal for industrial control systems, SCPI is better suited for test and measurement applications, and GPIB is well-suited for complex laboratory setups.
- Network Type: Modbus supports both serial and network communication, making it versatile for different types of setups. SCPI and GPIB are often used over Ethernet, USB, or GPIB interfaces.
- Command Complexity: SCPI commands are more human-readable and structured, which can be beneficial for complex instrument control and data acquisition tasks. Modbus commands are simpler and more efficient for real-time control.
- Interoperability: Modbus is widely adopted in industrial settings, ensuring compatibility with a broad range of devices and systems. GPIB is less common today but still used in specific environments where multiple instruments need to be controlled together.
Conclusion
In this article, we explored the Modbus, SCPI, and GPIB protocols, understanding their importance and use cases in industrial and testing environments. We also demonstrated how to implement these protocols on microcontrollers using Arduino and MicroPython. These examples provide a solid foundation for integrating Modbus, SCPI, and GPIB into your embedded projects, ensuring effective communication and control in various applications.