- Building Machines In Code
- Building Machines in Code – Part 2
- Building Machines In Code – Part 3
- Building Machines In Code – Part 4
- Building Machines In Code – Part 5
- Building Machines In Code – Part 6
- Building Machines In Code – Part 7
- Building Machines In Code – Part 8
- Building Machines In Code – Part 9
Post Stastics
- This post has 4678 words.
- Estimated read time is 22.28 minute(s).
Tooling
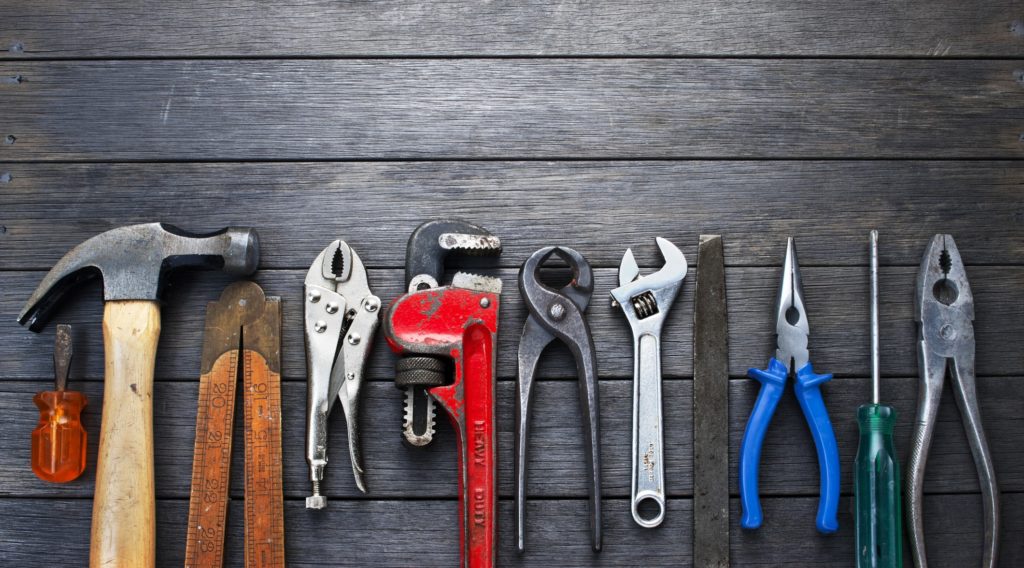
Hardware and software developers are tool makers by trade. Just like a machinist, software developers often need to develop their own tools for the job at hand. Sometimes these tools are simple scripts to automate a boring, or complicated task, or perhaps, a tool to fill a yet unfilled niche. Whatever the reason, tool making is quite common for some software developers. Hardware developers often have to develop tools such as assemblers, compilers, and programmers for the hardware systems they develop.
Since we don’t have an assembler for the Tiny-P, we will develop a simple assembler in this installment. Having an assembler will help us develop software more easily. Our assembler will have a couple of features to help us more easily write our assembly programs. Namely, labels and origin statements. Below is an example of a Tiny-P assembly language program for our assembler.
# Sample Tiny-P Assembler Program # Comments start with a hash symbol "#" ORG. 0 # Origin: starting address of program code start: LDA 0 # Load ACC with value stored in Mem[0] ADD 1 # Add value stored in Mem[1] to ACC STA 1 # Save result of Add in Mem[2] BRP start # If result is zero or greater return to start ...
Let’s dissect the assembly code above. The first is the “ORG.” command is an assembler directive. It tells the assembler where our program will start in program memory. If not provided, the assembler will default to an origin of 0. Next, we encounter the “start:” label. Labels are just named locations. Since our origin is set at 0 and LDA is the first instruction in our program start is equal to program address 0. Notice how the last instruction “BRP” (branch Positive) uses the “start” label instead of 0. Names are much easier to remember and keep track of in a longer assembly language program. Use them wisely and they will become your friend!
As you may have noticed in part-4 of this series, assembly language statements and machine code have an almost one-to-one correspondence. This makes developing a simple assembler rather easy. The approach I like to use is to define the assembly language grammar in EBNF (Extended Backus Naur Form) and yes, there is a BNF. These notations allow a developer to describe programming languages and protocols. Using the EBNF grammar I then head over to my favorite railroad-diagramming tool at: https://www.bottlecaps.de/rr/ui and generate a railroad diagram from my grammar:
PROG ::= STATEMENTS
no references
::= STATEMENT+
referenced by:
referenced by:
referenced by:
::= LABEL ':'
referenced by:
LABEL ::= [_a-zA-Z]+ [_a-zA-Z0-9]*
referenced by:
referenced by:
NUMBER ::= [0-9]+ [0-9]*
referenced by:
|